Note
Go to the end to download the full example code
Parse and Visualize HYDRAD Results#
This example demonstrates how to configure a simple hydrad simulation and read in and visualize the outputs as a function of time and field-aligned spatial coordinate.
import pathlib
import tempfile
import astropy.units as u
from astropy.visualization import ImageNormalize, LogStretch
from pydrad.configure import Configure
from pydrad.configure.data import get_defaults
from pydrad.configure.util import get_clean_hydrad, run_shell_command
from pydrad.parse import Strand
tmpdir = pathlib.Path(tempfile.mkdtemp()) # Change to wherever you want to save your clean HYDRAD copy
HYDRAD prints all results to the Results
directory in the main code
directory. These filenames are in the format profile{index}.{ext}
where index
is the timestep number and ext
can be one of the
following filetypes,
phy
– main physics results file containing temperature, density, etc.amr
– data related to the adaptive meshtrm
– equation terms at each grid cell
Parsing all of these files can be difficult. pydrad provides a convenient object, ~pydrad.parse.Strand, for easily accessing the data associated with a particular HYDRAD run.
First, we’ll setup a simple HYDRAD run and run the simulation. Grab a new copy of HYDRAD
hydrad_clean = tmpdir / 'hydrad-clean'
get_clean_hydrad(hydrad_clean, from_github=True)
We’ll start with the default configuration and setup a simple simulation for a 50 Mm loop lasting 500 s in which there are no heating events such that the loop is maintained in static equilibrium.
/home/docs/checkouts/readthedocs.org/user_builds/pydrad/envs/latest/lib/python3.11/site-packages/astropy/units/format/utils.py:219: UnitsWarning: The unit 'erg' has been deprecated in the VOUnit standard. Suggested: cm**2.g.s**-2.
warnings.warn(message, UnitsWarning)
To reduce the commputation time and memory footprint of our example simulation, we reduce the degree to which our spatial grid is refined. In general, this is something that should be done cautiously. See Bradshaw and Cargill (2013) for more details on appropriately resolving gradients in the transition region. This is an especially important consideration in impulsive heating scenarios.
Next, we’ll setup and run the simulation. We’ll run HYDRAD directly from Python, but this is easily done via the command line as well. This can take a few minutes.
c = Configure(config)
hydrad_results = tmpdir / 'steady-run'
c.setup_simulation(hydrad_results, hydrad_clean)
run_shell_command(hydrad_results / 'HYDRAD.exe')
WARNING: ../source/misc.cpp: In function ‘void GetConfigurationParameters(PARAMETERS*)’:
../source/misc.cpp:31:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
31 | fscanf( pFile, "%s", pParams->szOutputFilename );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenRangesFile(char*)’:
../../Radiation_Model/source/element.cpp:63:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
63 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:66:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
66 | fscanf( pFile, "%i", &NumTemp );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:67:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
67 | fscanf( pFile, "%i", &NumDen );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:76:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
76 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:85:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
85 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenAbundanceFile(char*)’:
../../Radiation_Model/source/element.cpp:105:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
105 | fscanf( pFile, "%i", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:121:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
121 | fscanf( pFile, "%i", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenEmissivityFile(char*)’:
../../Radiation_Model/source/element.cpp:152:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
152 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:154:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
154 | fscanf( pFile, "%i", &NumIons );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:162:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
162 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:166:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
166 | fscanf( pFile, "%i", &pSpecNum[i] );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:184:15: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
184 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenRatesFile(char*)’:
../../Radiation_Model/source/element.cpp:237:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
237 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:251:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
251 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp: In member function ‘void CRadiation::Initialise(char*)’:
../../Radiation_Model/source/radiation.cpp:92:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
92 | fscanf( pFile, "%s", buffer1 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:96:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
96 | fscanf( pFile, "%s", buffer2 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:99:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
99 | fscanf( pFile, "%s", buffer3 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:103:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
103 | fscanf( pFile, "%s", buffer4 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:106:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
106 | fscanf( pFile, "%i", &NumElements );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:117:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
117 | fscanf( pFile, "%s", buffer1 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:120:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
120 | fscanf( pFile, "%i", &(pZ[i]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp: In member function ‘void CRadiation::OpenRangesFile(char*)’:
../../Radiation_Model/source/radiation.cpp:156:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
156 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:159:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
159 | fscanf( pFile, "%i", &NumTemp );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:160:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
160 | fscanf( pFile, "%i", &NumDen );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:169:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
169 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:178:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
178 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp: In member function ‘void CPieceWiseFit::OpenPieceWiseFit(char*)’:
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:49:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
49 | fscanf( pInputFile, "%i", &iPolyOrder );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:51:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
51 | fscanf( pInputFile, "%i", &inumSD );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp: In member function ‘void CPieceWiseFit::GeneratePieceWiseFit(char*, char*)’:
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:92:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
92 | fscanf( pInputFile, "%i", &iPolyOrder );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:99:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
99 | fscanf( pInputFile, "%i", &inumSD );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:106:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
106 | fscanf( pInputFile, "%i", &inumPoints );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/source/file.cpp: In function ‘void ReadDouble(void*, double*)’:
../../Resources/source/file.cpp:24:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
24 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
[pydrad.configure.util]
INFO:
Calculating initial hydrostatic conditions...
Peak heating range = 7.6913e-04 -> 7.7090e-04 erg cm^-3 s^-1
Optimum peak heating rate = 7.6952e-04 erg cm^-3 s^-1
Writing initial conditions file...
Done!
[pydrad.configure.util]
WARNING: ../source/mesh.cpp: In member function ‘void CAdaptiveMesh::CreateInitialMesh()’:
../source/mesh.cpp:84:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
84 | fscanf( pFile, "%i", &iFileNumber );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/mesh.cpp:88:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
88 | fscanf( pFile, "%i", &Params.iNumberOfCells );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/mesh.cpp:112:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
112 | fscanf( pFile, "%i", &(CellProperties.iRefinementLevel) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/mesh.cpp:114:15: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
114 | fscanf( pFile, "%i", &(CellProperties.iUniqueID[j]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/eqns.cpp: In member function ‘void CEquations::Initialise()’:
../source/eqns.cpp:131:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
131 | fscanf( pFile, "%s", Params.Profiles );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/eqns.cpp:133:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
133 | fscanf( pFile, "%s", Params.GravityFilename );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../source/eqns.cpp:147:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
147 | fscanf( pFile, "%i", &iTemp );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Heating_Model/source/heat.cpp: In member function ‘void CHeat::GetHeatingData()’:
../../Heating_Model/source/heat.cpp:104:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
104 | fscanf( pConfigFile, "%i", &NumActivatedEvents );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp: In member function ‘void CIonFrac::Initialise(CIonFrac*, char*, PRADIATION)’:
../../Radiation_Model/source/ionfrac.cpp:49:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
49 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:52:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
52 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:55:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
55 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:58:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
58 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:61:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
61 | fscanf( pFile, "%i", &NumElements );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:74:15: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
74 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp:77:15: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
77 | fscanf( pFile, "%i", &(pZ[i]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp: In member function ‘void CIonFrac::ReadAllIonFracFromFile(void*)’:
../../Radiation_Model/source/ionfrac.cpp:199:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
199 | fscanf( (FILE*)pFile, "%i", &(pZ[i]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/ionfrac.cpp: In member function ‘void CIonFrac::ReadIonFracFromFile(void*, int)’:
../../Radiation_Model/source/ionfrac.cpp:216:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
216 | fscanf( (FILE*)pFile, "%i", &(pZ[i]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenRangesFile(char*)’:
../../Radiation_Model/source/element.cpp:63:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
63 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:66:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
66 | fscanf( pFile, "%i", &NumTemp );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:67:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
67 | fscanf( pFile, "%i", &NumDen );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:76:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
76 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:85:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
85 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenAbundanceFile(char*)’:
../../Radiation_Model/source/element.cpp:105:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
105 | fscanf( pFile, "%i", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:121:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
121 | fscanf( pFile, "%i", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenEmissivityFile(char*)’:
../../Radiation_Model/source/element.cpp:152:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
152 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:154:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
154 | fscanf( pFile, "%i", &NumIons );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:162:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
162 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:166:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
166 | fscanf( pFile, "%i", &pSpecNum[i] );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:184:15: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
184 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp: In member function ‘void CElement::OpenRatesFile(char*)’:
../../Radiation_Model/source/element.cpp:237:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
237 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/element.cpp:251:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
251 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp: In member function ‘void CRadiation::Initialise(char*)’:
../../Radiation_Model/source/radiation.cpp:92:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
92 | fscanf( pFile, "%s", buffer1 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:96:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
96 | fscanf( pFile, "%s", buffer2 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:99:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
99 | fscanf( pFile, "%s", buffer3 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:103:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
103 | fscanf( pFile, "%s", buffer4 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:106:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
106 | fscanf( pFile, "%i", &NumElements );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:117:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
117 | fscanf( pFile, "%s", buffer1 );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:120:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
120 | fscanf( pFile, "%i", &(pZ[i]) );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp: In member function ‘void CRadiation::OpenRangesFile(char*)’:
../../Radiation_Model/source/radiation.cpp:156:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
156 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:159:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
159 | fscanf( pFile, "%i", &NumTemp );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:160:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
160 | fscanf( pFile, "%i", &NumDen );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:169:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
169 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Radiation_Model/source/radiation.cpp:178:11: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
178 | fscanf( pFile, "%c", &buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp: In member function ‘void CPieceWiseFit::OpenPieceWiseFit(char*)’:
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:49:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
49 | fscanf( pInputFile, "%i", &iPolyOrder );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:51:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
51 | fscanf( pInputFile, "%i", &inumSD );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp: In member function ‘void CPieceWiseFit::GeneratePieceWiseFit(char*, char*)’:
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:92:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
92 | fscanf( pInputFile, "%i", &iPolyOrder );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:99:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
99 | fscanf( pInputFile, "%i", &inumSD );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/Utils/generatePieceWiseFit/source/piecewisefit.cpp:106:23: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
106 | fscanf( pInputFile, "%i", &inumPoints );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
../../Resources/source/file.cpp: In function ‘void ReadDouble(void*, double*)’:
../../Resources/source/file.cpp:24:7: warning: ignoring return value of ‘int fscanf(FILE*, const char*, ...)’ declared with attribute ‘warn_unused_result’ [-Wunused-result]
24 | fscanf( pFile, "%s", buffer );
| ~~~~~~^~~~~~~~~~~~~~~~~~~~~~~
[pydrad.configure.util]
/home/docs/checkouts/readthedocs.org/user_builds/pydrad/envs/latest/lib/python3.11/site-packages/astropy/units/format/utils.py:219: UnitsWarning: The unit 'erg' has been deprecated in the VOUnit standard. Suggested: cm**2.g.s**-2.
warnings.warn(message, UnitsWarning)
/home/docs/checkouts/readthedocs.org/user_builds/pydrad/envs/latest/lib/python3.11/site-packages/astropy/units/format/utils.py:219: UnitsWarning: The unit 'erg' has been deprecated in the VOUnit standard. Suggested: cm**2.g.s**-2.
warnings.warn(message, UnitsWarning)
/home/docs/checkouts/readthedocs.org/user_builds/pydrad/envs/latest/lib/python3.11/site-packages/astropy/units/format/utils.py:219: UnitsWarning: The unit 'erg' has been deprecated in the VOUnit standard. Suggested: cm**2.g.s**-2.
warnings.warn(message, UnitsWarning)
/home/docs/checkouts/readthedocs.org/user_builds/pydrad/envs/latest/lib/python3.11/site-packages/astropy/units/format/utils.py:219: UnitsWarning: The unit 'erg' has been deprecated in the VOUnit standard. Suggested: cm**2.g.s**-2.
warnings.warn(message, UnitsWarning)
INFO:
Processing the initial conditions...
Solving...
model-time elapsed = 9.7295e+00 s; dt = 9.6230e-03 s
wall-time elapsed = 3.0760e-01 s; ~ wall-time remaining = 1.5500e+01 s
wall-time/1000 steps = 3.0760e-01 s; wall-time/second = 3.1615e-02 s
refinement level = 6/6; total grid cells = 202
model-time elapsed = 1.5989e+01 s; dt = 6.0930e-03 s
wall-time elapsed = 6.3283e-01 s; ~ wall-time remaining = 1.9157e+01 s
wall-time/1000 steps = 3.2523e-01 s; wall-time/second = 3.9579e-02 s
refinement level = 6/6; total grid cells = 209
model-time elapsed = 2.5415e+01 s; dt = 9.2978e-03 s
wall-time elapsed = 1.0597e+00 s; ~ wall-time remaining = 1.9789e+01 s
wall-time/1000 steps = 4.2687e-01 s; wall-time/second = 4.1696e-02 s
refinement level = 6/6; total grid cells = 199
model-time elapsed = 3.4885e+01 s; dt = 9.3214e-03 s
wall-time elapsed = 1.3859e+00 s; ~ wall-time remaining = 1.8478e+01 s
wall-time/1000 steps = 3.2615e-01 s; wall-time/second = 3.9727e-02 s
refinement level = 6/6; total grid cells = 209
model-time elapsed = 4.4357e+01 s; dt = 9.3359e-03 s
wall-time elapsed = 1.7049e+00 s; ~ wall-time remaining = 1.7513e+01 s
wall-time/1000 steps = 3.1897e-01 s; wall-time/second = 3.8435e-02 s
refinement level = 6/6; total grid cells = 204
model-time elapsed = 5.3855e+01 s; dt = 9.3367e-03 s
wall-time elapsed = 2.0243e+00 s; ~ wall-time remaining = 1.6770e+01 s
wall-time/1000 steps = 3.1940e-01 s; wall-time/second = 3.7588e-02 s
refinement level = 6/6; total grid cells = 204
model-time elapsed = 6.3105e+01 s; dt = 9.2969e-03 s
wall-time elapsed = 2.4356e+00 s; ~ wall-time remaining = 1.6862e+01 s
wall-time/1000 steps = 4.1131e-01 s; wall-time/second = 3.8596e-02 s
refinement level = 6/6; total grid cells = 213
model-time elapsed = 6.6273e+01 s; dt = 2.9953e-03 s
wall-time elapsed = 2.8438e+00 s; ~ wall-time remaining = 1.8611e+01 s
wall-time/1000 steps = 4.0817e-01 s; wall-time/second = 4.2910e-02 s
refinement level = 6/6; total grid cells = 212
model-time elapsed = 7.5565e+01 s; dt = 9.2687e-03 s
wall-time elapsed = 3.3283e+00 s; ~ wall-time remaining = 1.8694e+01 s
wall-time/1000 steps = 4.8450e-01 s; wall-time/second = 4.4045e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 8.4994e+01 s; dt = 9.2844e-03 s
wall-time elapsed = 3.8330e+00 s; ~ wall-time remaining = 1.8715e+01 s
wall-time/1000 steps = 5.0465e-01 s; wall-time/second = 4.5097e-02 s
refinement level = 6/6; total grid cells = 209
model-time elapsed = 9.2869e+01 s; dt = 8.1498e-03 s
wall-time elapsed = 4.3149e+00 s; ~ wall-time remaining = 1.8916e+01 s
wall-time/1000 steps = 4.8194e-01 s; wall-time/second = 4.6462e-02 s
refinement level = 6/6; total grid cells = 200
model-time elapsed = 1.0052e+02 s; dt = 7.4367e-03 s
wall-time elapsed = 4.8143e+00 s; ~ wall-time remaining = 1.9133e+01 s
wall-time/1000 steps = 4.9937e-01 s; wall-time/second = 4.7895e-02 s
refinement level = 6/6; total grid cells = 207
model-time elapsed = 1.0992e+02 s; dt = 9.2432e-03 s
wall-time elapsed = 5.2865e+00 s; ~ wall-time remaining = 1.8761e+01 s
wall-time/1000 steps = 4.7214e-01 s; wall-time/second = 4.8094e-02 s
refinement level = 6/6; total grid cells = 199
model-time elapsed = 1.1930e+02 s; dt = 9.2350e-03 s
wall-time elapsed = 5.7596e+00 s; ~ wall-time remaining = 1.8379e+01 s
wall-time/1000 steps = 4.7315e-01 s; wall-time/second = 4.8277e-02 s
refinement level = 6/6; total grid cells = 196
model-time elapsed = 1.2611e+02 s; dt = 7.2317e-03 s
wall-time elapsed = 6.2934e+00 s; ~ wall-time remaining = 1.8659e+01 s
wall-time/1000 steps = 5.3373e-01 s; wall-time/second = 4.9905e-02 s
refinement level = 6/6; total grid cells = 221
model-time elapsed = 1.3551e+02 s; dt = 9.5554e-03 s
wall-time elapsed = 6.7794e+00 s; ~ wall-time remaining = 1.8235e+01 s
wall-time/1000 steps = 4.8603e-01 s; wall-time/second = 5.0029e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 1.4461e+02 s; dt = 8.8333e-03 s
wall-time elapsed = 7.2450e+00 s; ~ wall-time remaining = 1.7806e+01 s
wall-time/1000 steps = 4.6553e-01 s; wall-time/second = 5.0101e-02 s
refinement level = 6/6; total grid cells = 222
model-time elapsed = 1.5392e+02 s; dt = 9.1968e-03 s
wall-time elapsed = 7.5603e+00 s; ~ wall-time remaining = 1.6998e+01 s
wall-time/1000 steps = 3.1530e-01 s; wall-time/second = 4.9117e-02 s
refinement level = 6/6; total grid cells = 202
model-time elapsed = 1.6330e+02 s; dt = 9.2610e-03 s
wall-time elapsed = 7.8901e+00 s; ~ wall-time remaining = 1.6269e+01 s
wall-time/1000 steps = 3.2979e-01 s; wall-time/second = 4.8317e-02 s
refinement level = 6/6; total grid cells = 211
model-time elapsed = 1.7144e+02 s; dt = 7.2235e-03 s
wall-time elapsed = 8.2209e+00 s; ~ wall-time remaining = 1.5755e+01 s
wall-time/1000 steps = 3.3075e-01 s; wall-time/second = 4.7952e-02 s
refinement level = 6/6; total grid cells = 212
model-time elapsed = 1.8092e+02 s; dt = 9.3172e-03 s
wall-time elapsed = 8.5266e+00 s; ~ wall-time remaining = 1.5038e+01 s
wall-time/1000 steps = 3.0571e-01 s; wall-time/second = 4.7129e-02 s
refinement level = 6/6; total grid cells = 196
model-time elapsed = 1.9037e+02 s; dt = 9.2807e-03 s
wall-time elapsed = 8.8588e+00 s; ~ wall-time remaining = 1.4408e+01 s
wall-time/1000 steps = 3.3217e-01 s; wall-time/second = 4.6534e-02 s
refinement level = 6/6; total grid cells = 213
model-time elapsed = 1.9421e+02 s; dt = 4.0456e-03 s
wall-time elapsed = 9.1826e+00 s; ~ wall-time remaining = 1.4458e+01 s
wall-time/1000 steps = 3.2376e-01 s; wall-time/second = 4.7282e-02 s
refinement level = 6/6; total grid cells = 211
model-time elapsed = 2.0200e+02 s; dt = 8.0129e-03 s
wall-time elapsed = 9.4943e+00 s; ~ wall-time remaining = 1.4006e+01 s
wall-time/1000 steps = 3.1170e-01 s; wall-time/second = 4.7001e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 2.1142e+02 s; dt = 9.2711e-03 s
wall-time elapsed = 9.8108e+00 s; ~ wall-time remaining = 1.3391e+01 s
wall-time/1000 steps = 3.1651e-01 s; wall-time/second = 4.6403e-02 s
refinement level = 6/6; total grid cells = 202
model-time elapsed = 2.2083e+02 s; dt = 9.2336e-03 s
wall-time elapsed = 1.0144e+01 s; ~ wall-time remaining = 1.2823e+01 s
wall-time/1000 steps = 3.3301e-01 s; wall-time/second = 4.5934e-02 s
refinement level = 6/6; total grid cells = 213
model-time elapsed = 2.3019e+02 s; dt = 9.1875e-03 s
wall-time elapsed = 1.0471e+01 s; ~ wall-time remaining = 1.2273e+01 s
wall-time/1000 steps = 3.2729e-01 s; wall-time/second = 4.5489e-02 s
refinement level = 6/6; total grid cells = 209
model-time elapsed = 2.3952e+02 s; dt = 9.1823e-03 s
wall-time elapsed = 1.0790e+01 s; ~ wall-time remaining = 1.1735e+01 s
wall-time/1000 steps = 3.1932e-01 s; wall-time/second = 4.5051e-02 s
refinement level = 6/6; total grid cells = 207
model-time elapsed = 2.4203e+02 s; dt = 2.4089e-03 s
wall-time elapsed = 1.1146e+01 s; ~ wall-time remaining = 1.1881e+01 s
wall-time/1000 steps = 3.5586e-01 s; wall-time/second = 4.6054e-02 s
refinement level = 6/6; total grid cells = 228
model-time elapsed = 2.5152e+02 s; dt = 9.1384e-03 s
wall-time elapsed = 1.1466e+01 s; ~ wall-time remaining = 1.1328e+01 s
wall-time/1000 steps = 3.1990e-01 s; wall-time/second = 4.5588e-02 s
refinement level = 6/6; total grid cells = 206
model-time elapsed = 2.6089e+02 s; dt = 9.5380e-03 s
wall-time elapsed = 1.1795e+01 s; ~ wall-time remaining = 1.0811e+01 s
wall-time/1000 steps = 3.2897e-01 s; wall-time/second = 4.5212e-02 s
refinement level = 6/6; total grid cells = 206
model-time elapsed = 2.7030e+02 s; dt = 9.5660e-03 s
wall-time elapsed = 1.2105e+01 s; ~ wall-time remaining = 1.0287e+01 s
wall-time/1000 steps = 3.1017e-01 s; wall-time/second = 4.4786e-02 s
refinement level = 6/6; total grid cells = 198
model-time elapsed = 2.7970e+02 s; dt = 9.5389e-03 s
wall-time elapsed = 1.2416e+01 s; ~ wall-time remaining = 9.7797e+00 s
wall-time/1000 steps = 3.1100e-01 s; wall-time/second = 4.4392e-02 s
refinement level = 6/6; total grid cells = 202
model-time elapsed = 2.8908e+02 s; dt = 9.5329e-03 s
wall-time elapsed = 1.2736e+01 s; ~ wall-time remaining = 9.2924e+00 s
wall-time/1000 steps = 3.1919e-01 s; wall-time/second = 4.4056e-02 s
refinement level = 6/6; total grid cells = 204
model-time elapsed = 2.9847e+02 s; dt = 9.5375e-03 s
wall-time elapsed = 1.3061e+01 s; ~ wall-time remaining = 8.8188e+00 s
wall-time/1000 steps = 3.2562e-01 s; wall-time/second = 4.3760e-02 s
refinement level = 6/6; total grid cells = 208
model-time elapsed = 3.0781e+02 s; dt = 9.4345e-03 s
wall-time elapsed = 1.3371e+01 s; ~ wall-time remaining = 8.3486e+00 s
wall-time/1000 steps = 3.0934e-01 s; wall-time/second = 4.3438e-02 s
refinement level = 6/6; total grid cells = 198
model-time elapsed = 3.0979e+02 s; dt = 1.8934e-03 s
wall-time elapsed = 1.3710e+01 s; ~ wall-time remaining = 8.4182e+00 s
wall-time/1000 steps = 3.3966e-01 s; wall-time/second = 4.4257e-02 s
refinement level = 6/6; total grid cells = 219
model-time elapsed = 3.1457e+02 s; dt = 4.3378e-03 s
wall-time elapsed = 1.4027e+01 s; ~ wall-time remaining = 8.2684e+00 s
wall-time/1000 steps = 3.1639e-01 s; wall-time/second = 4.4590e-02 s
refinement level = 6/6; total grid cells = 203
model-time elapsed = 3.2309e+02 s; dt = 8.5466e-03 s
wall-time elapsed = 1.4338e+01 s; ~ wall-time remaining = 7.8510e+00 s
wall-time/1000 steps = 3.1177e-01 s; wall-time/second = 4.4379e-02 s
refinement level = 6/6; total grid cells = 200
model-time elapsed = 3.3099e+02 s; dt = 7.9878e-03 s
wall-time elapsed = 1.4676e+01 s; ~ wall-time remaining = 7.4942e+00 s
wall-time/1000 steps = 3.3763e-01 s; wall-time/second = 4.4341e-02 s
refinement level = 6/6; total grid cells = 216
model-time elapsed = 3.4025e+02 s; dt = 9.4452e-03 s
wall-time elapsed = 1.4988e+01 s; ~ wall-time remaining = 7.0374e+00 s
wall-time/1000 steps = 3.1237e-01 s; wall-time/second = 4.4052e-02 s
refinement level = 6/6; total grid cells = 200
model-time elapsed = 3.4875e+02 s; dt = 9.3853e-03 s
wall-time elapsed = 1.5306e+01 s; ~ wall-time remaining = 6.6384e+00 s
wall-time/1000 steps = 3.1774e-01 s; wall-time/second = 4.3889e-02 s
refinement level = 6/6; total grid cells = 207
model-time elapsed = 3.5815e+02 s; dt = 9.5709e-03 s
wall-time elapsed = 1.5620e+01 s; ~ wall-time remaining = 6.1863e+00 s
wall-time/1000 steps = 3.1405e-01 s; wall-time/second = 4.3613e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 3.6306e+02 s; dt = 4.9369e-03 s
wall-time elapsed = 1.5965e+01 s; ~ wall-time remaining = 6.0220e+00 s
wall-time/1000 steps = 3.4499e-01 s; wall-time/second = 4.3974e-02 s
refinement level = 6/6; total grid cells = 217
model-time elapsed = 3.7242e+02 s; dt = 9.5545e-03 s
wall-time elapsed = 1.6272e+01 s; ~ wall-time remaining = 5.5744e+00 s
wall-time/1000 steps = 3.0633e-01 s; wall-time/second = 4.3692e-02 s
refinement level = 6/6; total grid cells = 196
model-time elapsed = 3.7692e+02 s; dt = 4.3461e-03 s
wall-time elapsed = 1.6601e+01 s; ~ wall-time remaining = 5.4211e+00 s
wall-time/1000 steps = 3.2978e-01 s; wall-time/second = 4.4045e-02 s
refinement level = 6/6; total grid cells = 215
model-time elapsed = 3.8632e+02 s; dt = 9.3150e-03 s
wall-time elapsed = 1.6915e+01 s; ~ wall-time remaining = 4.9774e+00 s
wall-time/1000 steps = 3.1369e-01 s; wall-time/second = 4.3785e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 3.9174e+02 s; dt = 5.1060e-03 s
wall-time elapsed = 1.7270e+01 s; ~ wall-time remaining = 4.7724e+00 s
wall-time/1000 steps = 3.5455e-01 s; wall-time/second = 4.4084e-02 s
refinement level = 6/6; total grid cells = 227
model-time elapsed = 4.0118e+02 s; dt = 9.6066e-03 s
wall-time elapsed = 1.7580e+01 s; ~ wall-time remaining = 4.3303e+00 s
wall-time/1000 steps = 3.0984e-01 s; wall-time/second = 4.3820e-02 s
refinement level = 6/6; total grid cells = 198
model-time elapsed = 4.1018e+02 s; dt = 8.4934e-03 s
wall-time elapsed = 1.7915e+01 s; ~ wall-time remaining = 3.9229e+00 s
wall-time/1000 steps = 3.3571e-01 s; wall-time/second = 4.3676e-02 s
refinement level = 6/6; total grid cells = 213
model-time elapsed = 4.1948e+02 s; dt = 9.4870e-03 s
wall-time elapsed = 1.8229e+01 s; ~ wall-time remaining = 3.4991e+00 s
wall-time/1000 steps = 3.1362e-01 s; wall-time/second = 4.3456e-02 s
refinement level = 6/6; total grid cells = 204
model-time elapsed = 4.2386e+02 s; dt = 4.1776e-03 s
wall-time elapsed = 1.8547e+01 s; ~ wall-time remaining = 3.3315e+00 s
wall-time/1000 steps = 3.1787e-01 s; wall-time/second = 4.3757e-02 s
refinement level = 6/6; total grid cells = 204
model-time elapsed = 4.3164e+02 s; dt = 6.8167e-03 s
wall-time elapsed = 1.8863e+01 s; ~ wall-time remaining = 2.9875e+00 s
wall-time/1000 steps = 3.1576e-01 s; wall-time/second = 4.3700e-02 s
refinement level = 6/6; total grid cells = 203
model-time elapsed = 4.3462e+02 s; dt = 2.8103e-03 s
wall-time elapsed = 1.9190e+01 s; ~ wall-time remaining = 2.8869e+00 s
wall-time/1000 steps = 3.2782e-01 s; wall-time/second = 4.4154e-02 s
refinement level = 6/6; total grid cells = 214
model-time elapsed = 4.3673e+02 s; dt = 2.2891e-03 s
wall-time elapsed = 1.9501e+01 s; ~ wall-time remaining = 2.8251e+00 s
wall-time/1000 steps = 3.1056e-01 s; wall-time/second = 4.4652e-02 s
refinement level = 6/6; total grid cells = 203
model-time elapsed = 4.4605e+02 s; dt = 9.2978e-03 s
wall-time elapsed = 1.9804e+01 s; ~ wall-time remaining = 2.3953e+00 s
wall-time/1000 steps = 3.0301e-01 s; wall-time/second = 4.4398e-02 s
refinement level = 6/6; total grid cells = 194
model-time elapsed = 4.5312e+02 s; dt = 6.8158e-03 s
wall-time elapsed = 2.0143e+01 s; ~ wall-time remaining = 2.0840e+00 s
wall-time/1000 steps = 3.3934e-01 s; wall-time/second = 4.4455e-02 s
refinement level = 6/6; total grid cells = 216
model-time elapsed = 4.6246e+02 s; dt = 9.5104e-03 s
wall-time elapsed = 2.0458e+01 s; ~ wall-time remaining = 1.6605e+00 s
wall-time/1000 steps = 3.1430e-01 s; wall-time/second = 4.4236e-02 s
refinement level = 6/6; total grid cells = 201
model-time elapsed = 4.7187e+02 s; dt = 9.6121e-03 s
wall-time elapsed = 2.0764e+01 s; ~ wall-time remaining = 1.2378e+00 s
wall-time/1000 steps = 3.0605e-01 s; wall-time/second = 4.4003e-02 s
refinement level = 6/6; total grid cells = 196
model-time elapsed = 4.7804e+02 s; dt = 6.6761e-03 s
wall-time elapsed = 2.1104e+01 s; ~ wall-time remaining = 9.6961e-01 s
wall-time/1000 steps = 3.4001e-01 s; wall-time/second = 4.4147e-02 s
refinement level = 6/6; total grid cells = 221
model-time elapsed = 4.8754e+02 s; dt = 9.7215e-03 s
wall-time elapsed = 2.1430e+01 s; ~ wall-time remaining = 5.4788e-01 s
wall-time/1000 steps = 3.2589e-01 s; wall-time/second = 4.3955e-02 s
refinement level = 6/6; total grid cells = 208
model-time elapsed = 4.9197e+02 s; dt = 4.5820e-03 s
wall-time elapsed = 2.1759e+01 s; ~ wall-time remaining = 3.5529e-01 s
wall-time/1000 steps = 3.2947e-01 s; wall-time/second = 4.4229e-02 s
refinement level = 6/6; total grid cells = 211
Done!
[pydrad.configure.util]
To parse the results of a simulation, we create a ~pydard.parse.Strand object which holds information about the entire simulation.
s = Strand(hydrad_results)
print(s)
HYDrodynamics and RADiation (HYDRAD) Code
-----------------------------------------
Results path: /tmp/tmpudrlx3gl/steady-run
Time interval: [0.0 s, 500.008 s]
Number of profiles: 51
Loop length: 50.000 Mm
We can get basic information about the simulation such as the time and loop length
print(s.time)
print(s.loop_length)
[ 0. 10.0039 20.0028 30.0049 40.0001 50.0075 60.0027 70.0048
80.0039 90.0039 100.005 110.003 120.005 130.005 140.005 150.009
160.008 170.005 180.001 190.004 200.002 210.001 220.006 230.003
240.002 250.006 260.007 270.004 280.007 290.007 300.002 310.005
320.006 330.003 340.005 350.004 360.003 370.002 380.003 390.003
400.006 410.003 420.004 430.004 440.002 450.001 460.003 470.008
480.006 490.001 500.008 ] s
5000000000.0 cm
Indexing the ~pydrad.parse.Strand object, we get back a ~pydrad.parse.Profile object which holds information about a single snapshot of the simulation.
p = s[0]
print(p)
HYDRAD Timestep Profile
-----------------------
Filename: /tmp/tmpudrlx3gl/steady-run/Results/profile0.phy
Timestep #: 0
The ~pydrad.parse.Profile object also holds the thermodynamic quantities as a function of spatial coordinate at this particular timestep. Note that the quantity at each time step is stored as a separate 1D array as the spatial grid from one time step to the next need not be the same due to the use of the adaptively refined grid. Note that each profile is a ~astropy.units.Quantity and can be easily converted to any compatible unit.
print(p.electron_temperature)
print(p.ion_density)
print(p.velocity)
[ 20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20798.6104 19213.3114
16440.7517 16556.2916 17438.2987 19170.8913 21267.7631
23263.7091 25855.9661 29890.9259 36463.4647 48845.2523
79874.1744 143781.469 180118.62 206425.8 236101.268
267714.727 303543.35 341825.871 385390.725 432009.889
485124.497 542009.211 607042.572 676869.65 756598.148
841786.338 910176.801 967717.34 1017516.85 1061437.44
1100695.86 1136137.09 1168374.93 1197871.1 1224982.73
1249992.32 1273127.49 1294574.58 1314488.09 1332997.66
1350212.95 1366227.74 1381122.67 1394967.52 1407822.95
1419742.02 1430770.94 1440950.5 1450316.9 1458901.95
1466733.46 1473835.92 1480230.83 1485936.97 1490970.67
1495346.01 1499074.98 1502167.6 1504632.09 1506474.91
1507700.83 1508310.45 1508306.1 1507700.98 1506475.15
1504632.44 1502168.05 1499075.52 1495346.66 1490971.42
1485937.83 1480231.79 1473836.99 1466734.64 1458903.24
1450318.31 1440952.03 1430772.46 1419743.51 1407824.45
1394969.04 1381124.24 1366229.32 1350214.55 1332999.28
1314489.91 1294576.5 1273129.5 1249994.4 1224984.9
1197873.35 1168377.29 1136139.63 1100698.66 1061440.54
1017520.29 967721.312 910181.426 841791.994 756605.419
676879.019 607054.645 542024.609 485144.328 432035.478
385423.697 341868.265 303597.995 267785.062 236192.295
206543.39 180271.102 144010.059 80338.6471 48992.318
36529.7638 29915.7872 25881.2589 22147.3636 19226.0896
16976.5598 16433.9347 19211.6163 20800.475 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. 20000. 20000. 20000.
20000. 20000. ] K
[1.79790804e+13 1.57162717e+13 1.37389400e+13 1.20111886e+13
1.05015025e+13 9.18233680e+12 8.02961910e+12 7.02231377e+12
6.14204058e+12 5.37274084e+12 4.70038519e+12 4.11271798e+12
3.59903359e+12 3.14998090e+12 2.75739216e+12 2.41413354e+12
2.11397432e+12 1.85147267e+12 1.62187581e+12 1.42103272e+12
1.24531791e+12 1.09156485e+12 9.57007779e+11 8.39230902e+11
7.36124010e+11 6.45843730e+11 5.66779694e+11 4.97524985e+11
4.36850348e+11 3.83681658e+11 3.37080266e+11 2.96225823e+11
2.60401301e+11 2.28979905e+11 2.01413650e+11 1.77223395e+11
1.55990132e+11 1.37347388e+11 1.20064836e+11 1.21884349e+11
1.35945915e+11 1.31751589e+11 1.23095759e+11 1.10199129e+11
9.81626547e+10 8.90392578e+10 7.94861081e+10 6.82314258e+10
5.55161363e+10 4.11478222e+10 2.49951898e+10 1.38660042e+10
1.10579366e+10 9.64084088e+09 8.42016448e+09 7.41658492e+09
6.53044933e+09 5.78778615e+09 5.12033370e+09 4.55378229e+09
4.03873566e+09 3.59731940e+09 3.19127336e+09 2.84007253e+09
2.51501772e+09 2.23324745e+09 2.04304830e+09 1.90244084e+09
1.79258904e+09 1.70351338e+09 1.62933085e+09 1.56630315e+09
1.51191838e+09 1.46441400e+09 1.42250960e+09 1.38524855e+09
1.35189901e+09 1.32189006e+09 1.29476861e+09 1.27016986e+09
1.24779651e+09 1.22740336e+09 1.20878632e+09 1.19177417e+09
1.17622219e+09 1.16200722e+09 1.14902425e+09 1.13718295e+09
1.12640518e+09 1.11662351e+09 1.10777982e+09 1.09982380e+09
1.09271198e+09 1.08640686e+09 1.08087625e+09 1.07609272e+09
1.07203312e+09 1.06867823e+09 1.06601245e+09 1.06402357e+09
1.06270258e+09 1.06204632e+09 1.06205103e+09 1.06270247e+09
1.06402340e+09 1.06601221e+09 1.06867792e+09 1.07203273e+09
1.07609226e+09 1.08087571e+09 1.08640624e+09 1.09271128e+09
1.09982301e+09 1.10777894e+09 1.11662255e+09 1.12640413e+09
1.13718180e+09 1.14902312e+09 1.16200612e+09 1.17622109e+09
1.19177307e+09 1.20878516e+09 1.22740218e+09 1.24779531e+09
1.27016862e+09 1.29476711e+09 1.32188841e+09 1.35189723e+09
1.38524660e+09 1.42250749e+09 1.46441166e+09 1.51191579e+09
1.56630013e+09 1.62932720e+09 1.70350891e+09 1.79258351e+09
1.90243356e+09 2.04303844e+09 2.23323291e+09 2.51499392e+09
2.84003337e+09 3.19120971e+09 3.59721653e+09 4.03856919e+09
4.55351017e+09 5.11989191e+09 5.78706260e+09 6.52926540e+09
7.41462490e+09 8.41690227e+09 9.63532791e+09 1.10485494e+10
1.38439363e+10 2.48504665e+10 4.10241755e+10 5.54154394e+10
6.81746940e+10 7.94093741e+10 9.38936435e+10 1.09887654e+11
1.27468944e+11 1.36004604e+11 1.21896682e+11 1.20055404e+11
1.37349035e+11 1.55992002e+11 1.77225520e+11 2.01416065e+11
2.28982651e+11 2.60404424e+11 2.96229376e+11 3.37084309e+11
3.83686260e+11 4.36855588e+11 4.97530954e+11 5.66786493e+11
6.45851479e+11 7.36132842e+11 8.39240972e+11 9.57019262e+11
1.09157795e+12 1.24533285e+12 1.42104977e+12 1.62189528e+12
1.85149490e+12 2.11399969e+12 2.41416252e+12 2.75742527e+12
3.15001872e+12 3.59907681e+12 4.11276736e+12 4.70044163e+12
5.37280536e+12 6.14211434e+12 7.02239811e+12 8.02971554e+12
9.18244709e+12 1.05016286e+13 1.20113329e+13 1.37391051e+13
1.57164605e+13 1.79792642e+13] 1 / cm3
[0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.
0. 0. 0. 0. 0. 0. 0. 0. 0. 0.] cm / s
The ~pydrad.parse.Strand object also holds an additional ~pydrad.parse.Profile object for the initial conditions,
print(s.initial_conditions)
HYDRAD Timestep Profile
-----------------------
Filename: /tmp/tmpudrlx3gl/steady-run/Initial_Conditions/profiles/initial.amr.phy
Timestep #: 0
The ~pydrad.parse.Strand object can also be indexed in more complex ways in the same manner as a list or array to get the simulation only over a particular time range or at a particular time cadence. As an example, we can grab only the first 5 time steps or every other time step.
print(s[:5])
print(s[::2])
HYDrodynamics and RADiation (HYDRAD) Code
-----------------------------------------
Results path: /tmp/tmpudrlx3gl/steady-run
Time interval: [0.0 s, 40.0001 s]
Number of profiles: 5
Loop length: 50.000 Mm
HYDrodynamics and RADiation (HYDRAD) Code
-----------------------------------------
Results path: /tmp/tmpudrlx3gl/steady-run
Time interval: [0.0 s, 500.008 s]
Number of profiles: 26
Loop length: 50.000 Mm
The simplest way to visualize HYDRAD output is to look at a single time step as a function of field-aligned coordinate. The ~pydrad.parse.Profile object provides a simple quicklook method for this,
s[0].peek()
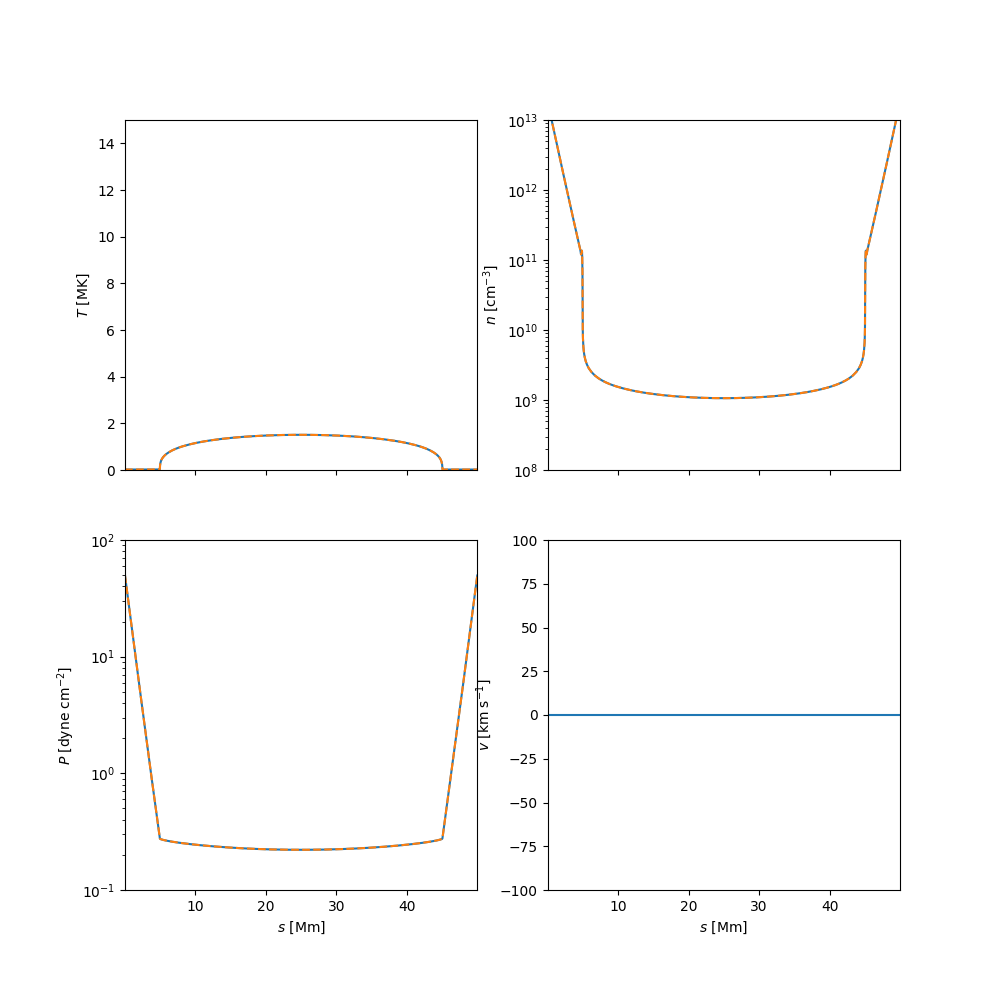
Similarly, we can call this method on a ~pydrad.parse.Strand to overplot every profile at each time step, where the time step is mapped to the colormap. For additional information about what arguments can be passed in here, see the ~pydrad.visualize.plot_profile documentation.
s.peek()
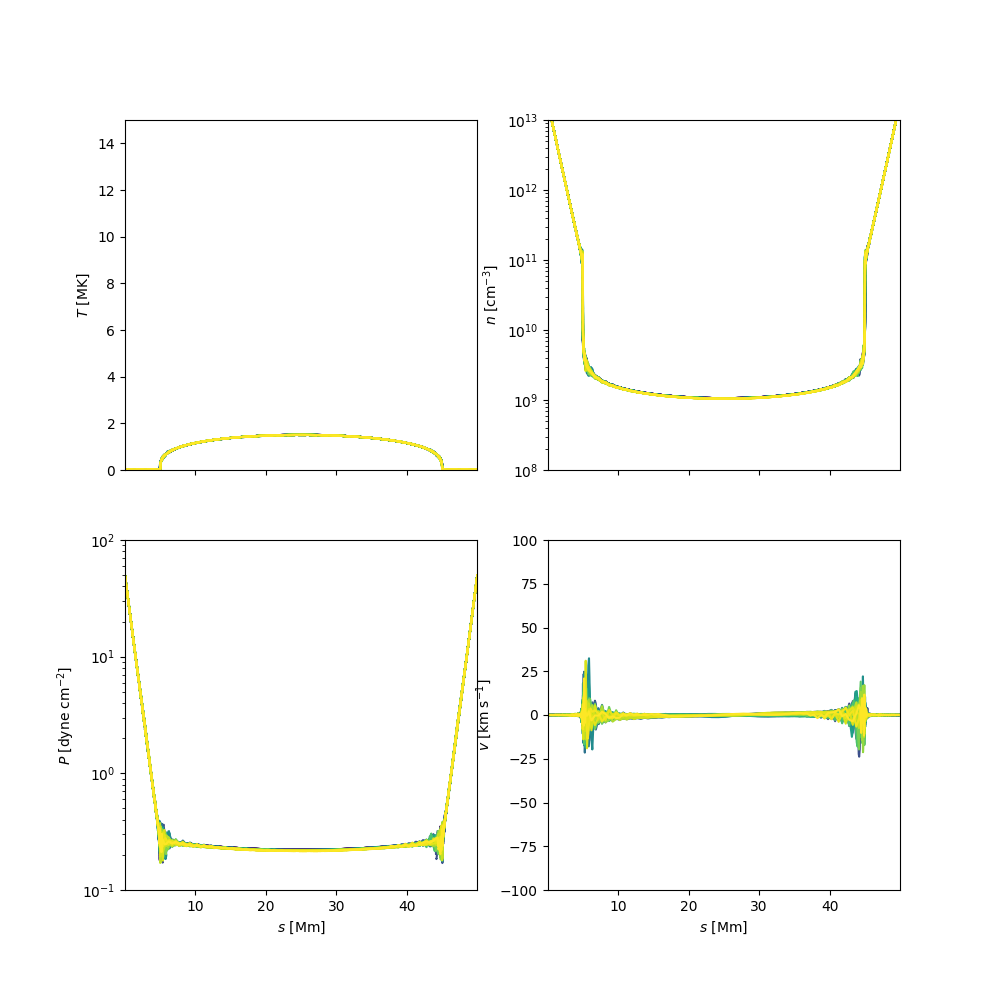
Often we want to visualize the whole loop as a function of time as a “time-distance” plot. The ~pydrad.parse.Strand object provides a convenience method for this visualization as well. Because of the differences in spatial grids between time steps, the quantities are reinterpolated onto a uniform grid of specified resolution, in this case 0.5 Mm, before plotting.
s.peek_time_distance('electron_temperature', 0.5*u.Mm)
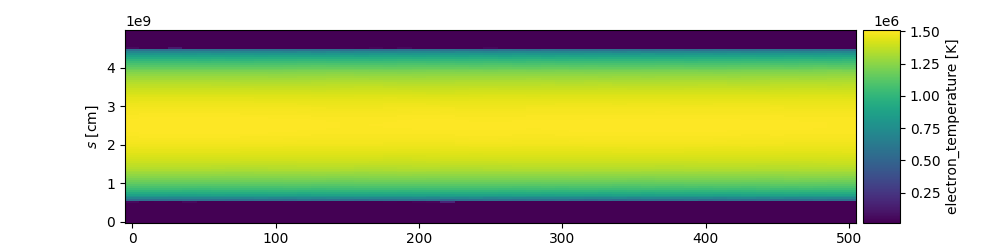
This quicklook function is flexible enough to visualize multiple quantities on different colormaps
s.peek_time_distance(
['electron_temperature', 'electron_density', 'velocity'],
0.5*u.Mm,
cmap={'velocity': 'RdBu_r'},
labels={'electron_temperature': r'$T_e$',
'electron_density': r'$n_e$'},
norm={'electron_density': ImageNormalize(vmin=1e8, vmax=1e12, stretch=LogStretch())},
time_unit='h',
space_unit='Mm',
)
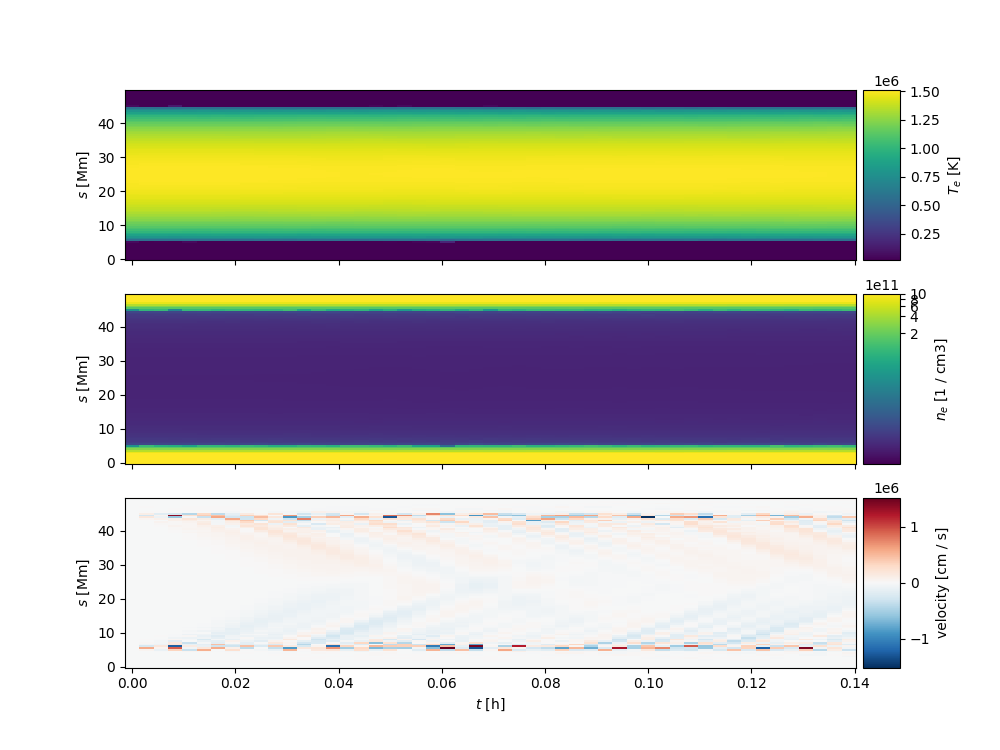
Total running time of the script: (0 minutes 42.958 seconds)